|  |
tutorial |
perform user functions on web applications
Java Server Pages (JSP) combine HTML, JavaScript,
and Java code and provide a simple programming method to display and
dynamically update content on Web pages. They lets you create complex
client/server applications for the Web through the dynamic substitutions
within an HTML page.
The following examples show how to implement some basic function with
JSP pages.
NOTE: for old versions before the 4.0, it's very important to note that if the application server
connects to the database indirectly through a HTTP / RMI
interface all java API methods with the "framework"
input argument don't work properly. In this case (iit is a best practice
to do it always) you MUST use the JRClient class methods to perform the operations.
doLogin.jsp
This JSP page performs the login to OBEROn for the
username and password passed as input parameters from the login page
and generates the application menu with all commands available for
the framework user.
The html code for the navigation menu is stored into a session attribute
and it doesn't change until the user re-logins to the system. Moreover,
the menu-command labels are translated to the browser language if
their translations are defined into the OBEROn dictionary.
It is very important to note the
setting: framework.setRenderingEngine("HTML");
this parameter included into the framework informs the generic user interface level that current user is connecting through a web interface; you can implement some filters to enable / disable application functionality according to this fact.
<%@include file
="library.jsp"%>
<% |
|
session.setAttribute("RootPath",request.getContextPath());
// Read the user account parameters from the login page
String sUser= getInputParameter("user",request);
String sPwd = getInputParameter("password",request);
String sForwardPage= getInputParameter("errorpage",request);
if ( sForwardPage.length()==0
) { sForwardPage="/index.jsp?user="+encodeURL(sUser);
}
if ( sUser.trim().length()>0
)
try {
|
|
|
// Try to open the framework
with the input userName and Password
Framework framework = new Framework( sUser
, sPwd );
framework.setRenderingEngine("HTML");
framework.link();
// Save the framework into the session
Application.setFramework(session,framework);
// Load the application
parameters from the main menu features
loadApplicationParameters(session);
// Set session attributes for
dictionary menu and common sections
session.setAttribute("MenuSection",getAppParam("dictionary_menu_section"));
session.setAttribute("CommonSection",getAppParam("dictionary_common_section"));
// Set the language
based on the browser language if "use_browser_language option" is enabled
if ( use_browser_language || framework.getLanguage().length()==0 ) {
|
|
|
|
framework.setLanguage( translateBrowserLanguageToOberonLanguage(
getBrowserLanguage(request) ) );
|
|
|
}
// Create the
application menu for the framework user (will be stored
into session attribute "Menu")
createMenu( application_main_menu
, session );
sForwardPage = "home.jsp";
|
|
} catch
(Exception e) { |
|
|
/*... do something
when the user and/or password are not valid ....
go forward to the error page */
setErrorMessage(session,"Login
for user '"+sUser+"'
FAILED" );
session.removeAttribute(Arg.Framework); |
|
}
|
%>
<jsp:forward page ="<%= sForwardPage %>"/>
|
|
menu.jsp
This JSP page is an include page (the header page);
it shows the navigation menu extracted from the session attribute.
<%@include
file ="library.jsp"%>
<% |
|
// Read the input parameters
String sTitle= getInputParameter("title",request);
String sPageTitle = getInputParameter("pagetitle",request);
if
( sPageTitle.length()==0
) { sPageTitle=sTitle; }
boolean bPopUp=getInputParameter("popup",request).equals("true");
boolean bHideBar=getInputParameter("hidebar",request).equals("true");
Framework framework = Application.getFramework(session);
String sUserName = framework.getUserName(); |
%>
<HTML>
<head>
|
|
<title><%=
sPageTitle %></title>
<meta http-equiv="Content-Type" content="text/html;
charset=UTF-8" />
<link rel="stylesheet" type="text/css"
href="style.css" /> |
</head>
<body topmargin="0"
marginwidth="0" marginheight="0">
<%
if
(!bPopUp) {
%> |
|
<script
src="menu.js" type="text/javascript"></script>
<!-- TOP BAR -->
<div class=menu-title><nobr><%=
sTitle %></nobr></div>
<div class=menu-subtitle><nobr><%=
getInputParameter("subtitle",request)
%></nobr></div>
<%
if
(!sUserName.equals(anonymous_user_name))
{ %>
|
|
<div
class=menu-user><nobr><b><%=
sUserName
%></b></nobr></div>
|
|
<%
} %>
<%
if
(!bHideBar) {
%>
|
|
<!--
Print the navigation menu on the html page ->
<div id="menu"> |
|
|
<!-- MENU BAR -->
<ul id="nav" >
<%= session.getAttribute("Menu")
%>
</ul>
<!-- OBJECT- Contextual
MENU -->
<ul class="select">
<%= createObjectMenu(session)
%>
</ul> |
|
</div> |
|
<%
} %> |
<%
} else { %>
<!--.... Show Title
/ SubTitle only ->
<%
}
//
Show error messages if any
String sMessage = getErrorMessage(session);
if
( sMessage.length()>0 ) { %>
|
|
<table align=center><tr><td><%=
sMessage%></td></tr></table> |
<%
} %>
</body>
|
|
edit.jsp
The following JSP page can perform both the object
creation and the object data update (if the input ID is passed as
input parameter). It also manages both the user interface for data
input (createHTMLForm) and the database update process (saveFormData).
These two methods are defined into the "forms.jsp" include
page. The forms.jsp page represents the HTML implementation for the
form administrative objects: in other words it generates the HTML
code based on the form parameters and on the form-item parameters,
included all the AJAX calls needed to load/refresh the field ranges
and to validate the field-item values.
<%@include
file ="library.jsp"%>
<% |
|
// Get the object
id as input parameter
String sID = request.getParameter("id");
boolean bCreateNew;
boolean bClone = false;
ObjectObj object;
Framework framework = Application.getFramework(session);
if ( sID==null
|| sID.length()==0 || getInputParameter("create",request).equals("true"))
{ |
|
|
// Create a new
object
bCreateNew=true;
object = new ObjectObj();
// Remove the "Object"
from session to hide the object contextual menu
session.removeAttribute("Object");
|
|
} else
{ |
|
|
// Open the object
and read its properties
bCreateNew=false;
sID=sID.trim();
if ( getInputParameter("clone",request).equals("true")
) { bClone=true; }
object = ObjectObj.open(sID,framework);
// Add the "Object"
to session to show the object contextual menu
session.setAttribute("Object",object);
|
|
}
// Get the create/edit Form name
as input parameter
String sFormName = getInputParameter("form",request);
if ( sFormName.length()==0
) { |
|
|
// When the form
is not passed as input parameter try to get it from the
object class
Class objclass = Class.open(object.getClassName(),framework,null);
sFormName=objclass.getDefaultForm(true); |
|
}
Form form = Form.open(sFormName,framework,null);
// Save the object data or create
it
String sForwardPage = saveFormData( bCreateNew?"edit.jsp":""
, object , form , |
|
|
convertRequest(request)); |
|
if ( sForwardPage.length()>0 ) { |
|
|
//
A new object is succesfully created - go forward to the
object detail page
%>
<script language=javascript>document.location.href="<%=
sForwardPage %>";</script>
<%
return; |
|
} |
//
Define title and subtitle for the menu bar
String sTitle = Dictionary.getKey(dictSection,sFormName+(bClone?"_clone":""),framework);
String sSubTitle = "";
if ( sID!=null
&& sID.length()>0
) { |
|
if
(bCreateNew) { |
|
|
//
Clone an existing object
object =ObjectObj.open(sID,framework);
object.resetID(); |
|
}
sSubTitle=sTitle;
sTitle= getClassNameRevision(object,session);
String commonSection = getAppParam("dictionary_common_section");
|
}
%>
<jsp:include page ="menu.jsp" flush="true"> |
|
<jsp:param name="title"
value="<%= sTitle
%>" />
<jsp:param name="subtitle" value="<%=
sSubTitle %>" />
<jsp:param name="popup" value="<%=
(request.getParameter("popup")!=null?"true":"false")%>"
/> |
</jsp:include>
<%@include file ="forms.jsp" %>
<!--
Print the html form with object field values->
<BODY> |
|
<br>
<%= createHTMLForm( getRootPath(session)+applicationPath+"edit.jsp"
, object , form , convertRequest(request))
%>
<br> |
<%
if
( sID!=null
) {
%>
|
|
<script
language="javascript"> |
|
|
function
cloneObject(form) {
document.location.href="edit.jsp?id=<%=
encodeURL(sID) %>&clone=true&form="+form;
}
function deleteObject(fwd) {
doObjectDestroy('<%=
encodeURL(sID)%>',"<%=
Dictionary.getKey (commonSection , "RemoveObjectMessage" , framework)
%>",fwd);
} |
|
</script> |
<%
}
%>
</BODY>
</HTML>
|
|
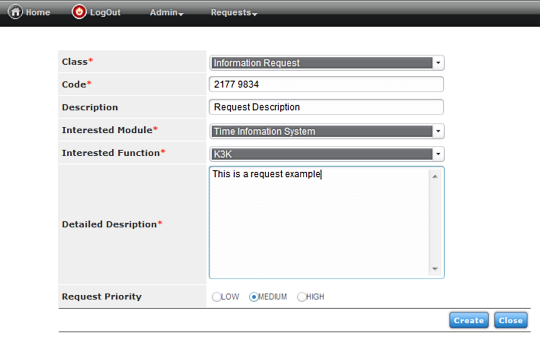
search.jsp
The search.jsp is similar to the edit.jsp: it manages
the user interface for the query input filters (createHTMLSearchForm);
this method is also defined into the "forms.jsp" include
page. It requires the search form name as input value and shows the
HTML code based on this form parameters and on its item parameters.
<%@include
file ="library.jsp"%>
<% |
|
// Get the search
Form name as input parameter
String sFormName = getInputParameter("form"
,request);
if ( sFormName.length()==0)
{ |
|
|
out.println("NO
INPUT FORM");
return; |
|
}
Framework framework = Application.getFramework(session);
Form form = Form.open(sFormName,framework,null); |
session.removeAttribute("view");
session.removeAttribute("sequence");
session.removeAttribute("Object");
// Define title and subtitle for the menu bar
String sTitle =Dictionary.getKey(dictSection,sFormName,framework);
String sSubTitle = "";
%>
<jsp:include page ="menu.jsp" flush="true"> |
|
<jsp:param name="title"
value="<%= sTitle
%>" />
<jsp:param name="subtitle" value="<%=
sSubTitle %>" /> |
</jsp:include>
<%@include file ="forms.jsp" %>
<!-- Print the html search form ->
<BODY> |
|
<br>
<%= createHTMLSearchForm(
"search_results.jsp"
, form , convertRequest(request)) %>
<br> |
</BODY>
</HTML> |
|
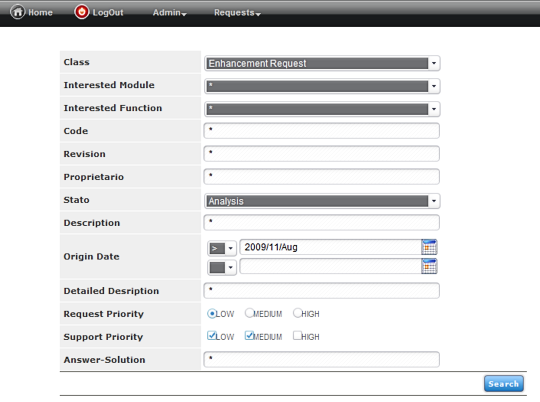
search_results.jsp
This JSP page executes the search query based on the filters defined
into the search.jsp. It employs the searchObjects method included
into the "forms.jsp" page. In addition, you can apply a
view (applyView) to the query results to extract and show several
object properties.
<%@include
file ="library.jsp"%>
<% |
|
// Get the search
Form name as input parameter
String sFormName = getInputParameter("form"
,request);
if ( sFormName.length()==0)
{ |
|
|
out.println("NO
INPUT FORM");
return; |
|
}
Framework framework = Application.getFramework(session);
Form form = Form.open(sFormName,framework,null);
String formHTMLName=getFormHTMLName(form);
// Execute the search query
try {
%><script
language=javascript><%
|
|
|
clearSearchResults( formHTMLName , session
);
out.println( searchObjects( form , convertRequest(request) ) ); |
|
%></script><%
} catch
(Exception e) { |
|
|
out.println("<font
color=red>"+e.getMessage()+"</font>"); |
|
}
// Get user views
Vector vViews = listUserViews(framework);
// Apply a view to search results
String sView = getInputParameter( "view"
,request);
View view ;
if (!sView.equals("")
&& vViews.indexOf(sView)>=0)
{
|
|
|
view = applyView(sView , formHTMLName ,
session); |
|
} |
// Define
title and subtitle for the menu bar
String sTitle = Dictionary.getKey(dictSection,sFormName,framework);
%>
<jsp:include page ="menu.jsp" flush="true">
|
|
<jsp:param name="title"
value="<%= sTitle
%>" /> |
</jsp:include>
<%@include file ="forms.jsp" %>
<!-- Print the result objects ->
<BODY>
<table> |
|
<% |
|
|
// Show the result
object properties; this is a simple output, you can apply
a more complex formatter
Vector vResults = getSearchResults( formHTMLName ,
session );
for (int
i=0;i<vResults.size();i++)
{ %> |
|
|
|
<tr><td><%=
vResults.elementAt(i)
%></td></tr><% |
|
|
} %> |
</table>
</BODY>
</HTML> |
|
fileget.jsp
This JSP page shows how to download from OBEROn
the attached files for a specific object instance. It extracts the
file properties and displays on a html table their download links.
The download process is performed with the JRFileGetServlet.
<jsp:include page ="menu.jsp" flush="true"/>
<BODY>
<table>
<% |
|
// Get the object
ID as input parameter
String sID = request.getParameter("id").trim(); |
|
Framework framework = Application.getFramework(session);
// Retrieve the
object attached file properties
ObjectObj object = ObjectObj.open(sID,false,framework);
Vector vFiles = object.getFiles(framework);
// Print the list of file download
links
for (int
i=0;i<vFiles.size();i++)
{ |
|
|
ObjectFile fFile = (ObjectFile)
vFiles.elementAt(i); %> |
|
|
<tr> |
|
|
|
<td><a href="javascript:fileGet('<%=
fFile.getFileType()
%>','<%=
fFile.getName()
%>')">
<%= fFile.getName()
%></a></td>
<td><%= fFile.getFileSize()
%> bytes</td>
<td><% if
(fFile.getLocker().length()>0)
{ out.print("Locked by"+fFile.getLocker());}
%></td> |
|
|
</tr><% |
|
} |
%>
</table>
<!--
Call the fileget servlet to download the file from OBEROn
->
<script language=javascript> |
|
function
fileGet(filetype,filename) { |
|
|
window.open("servlet/JRFileGetServlet?id=<%= encodeURL(sID) %> |
|
|
|
&filetype="+filetype+"&filename="+filename); |
|
}
|
|
</script>
</BODY>
</HTML> |
|
JRFileGetServlet
input parameters |
|
id
|
object id |
filetype
|
the attached file
type |
filename
|
the file name |
lock
|
"true"
= lock the object |
|
|
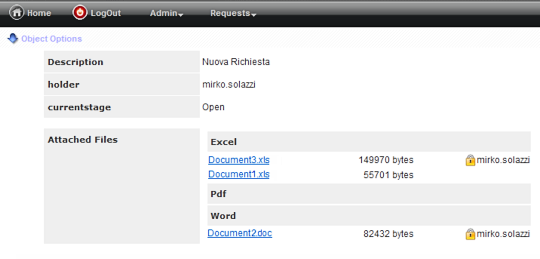
fileput.jsp
This JSP page shows how to upload a file to OBEROn
and attach it to a specific object instance. The file is sent to the
JRFilePutServlet togheter
with other parameters as a "multipart/form-data" request
format.
<jsp:include page ="menu.jsp" flush="true"/>
<BODY>
<% |
|
// Get the object
ID as input parameter
String sID = request.getParameter("id").trim();
String sForwardPage = "fileget.jsp?id="
+ encodeURL(sID);
Framework framework = Application.getFramework(session); |
%>
<!-- Call
the fileput servlet in post mode to upload the file to
OBEROn ->
<FORM action="servlet/JRFilePutServlet"
name=putForm method=post enctype="multipart/form-data"
> |
|
<input name="id"
type="hidden" value="<%= encodeURL(sID)
%>">
<input name="forward" type="hidden"
value="<%=
sForwardPage
%>">
<table> |
|
|
<tr> |
|
|
|
<td><%=
Dictionary.getKey("*","File",framework)%></td>
<td><input name="fileput" type=file></td> |
|
|
</tr> |
|
|
<tr> |
|
|
|
<td><%=
Dictionary.getKey("*","FileName",framework)%></td>
<td><input name="filename" type=text
></td> |
|
|
</tr> |
|
|
<tr> |
|
|
|
<td><%=
Dictionary.getKey("*","FileType",framework)%></td>
<td> |
|
|
|
|
<select
name="filetype">
<option value="Word" ><%=
Dictionary.getKey("*","Word",framework)</option>
<option value="Excel" ><%= Dictionary.getKey("*","Excel",framework)</option>
</select>
|
|
|
|
</td> |
|
|
</tr> |
|
|
<tr> |
|
|
|
<td><%=
Dictionary.getKey("*","Options",framework)%></td>
<td> |
|
|
|
<input name="unlock"
type="checkbox" value="true" />
<label for="unlock"><%=Dictionary.getKey("*","UnLock",framework)%></label>
<input name="overwrite" type="checkbox"
value="true" />
<label for="overwrite"><%=Dictionary.getKey("*","Overwrite",framework)%></label>
<input type="submit" value="<%=Dictionary.getKey("*","Send",framework)%>"/> |
|
|
|
</td> |
|
|
</tr> |
|
</table>
|
</FORM>
</BODY>
</HTML> |
|
JRFilePutServlet
input parameters |
|
id
|
object id |
fileput
|
the multipart request
attached file data |
filetype
|
the file type |
filename
|
the file name to
set |
unlock
|
"true"
= unlock the object |
overwrite
|
"true"
if you want remove all files stored for the same
filetype |
forward
|
the JSP forward
page |
|
|
lifecycle.jsp
This JSP page loads the object's lifecycle and
generate its graphical representation. The user will be able to
progress or regress the status and to validate/refuse the path validations.
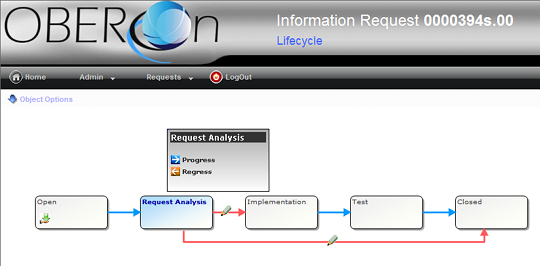
navigate.jsp
Shows the object's navigation tree: links can be
filtered selecting the linktype and the direction.
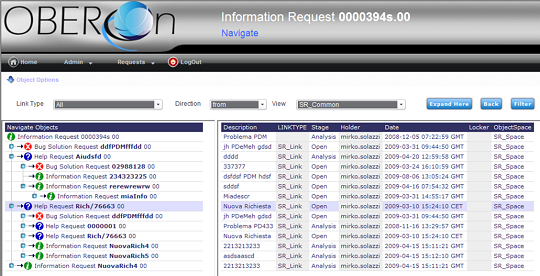
spacetree.jsp
Visualizes the object's links by a spacial tree
representation.
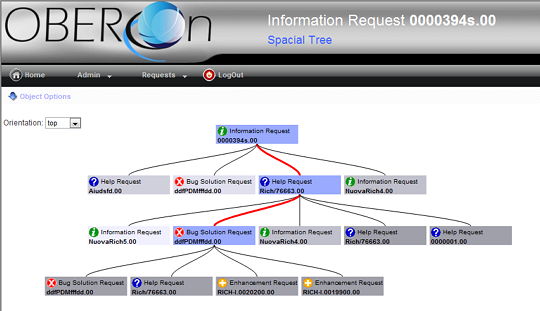
testgraph.jsp
This is a simple example that show how to generate
charts using the OBEROn internal chart engine. The JRDrawGraphServlet
is invoked passing to it the name of graph administrative object representing
the chart style and the xml data representing the plot values. The
servlet can also be called by a AJAX request.
<jsp:include page ="menu.jsp" flush="true"/>
<BODY>
<% |
|
String
sGraphName = request.getParameter("graph").trim();
Framework framework = Application.getFramework(session);
// Define the chart series values
GraphValues graphicValues = new
GraphValues();
graphicValues.addDataLabel(graphicValues.new
DataLabel("January",0,true));
graphicValues.addDataLabel(graphicValues.new
DataLabel("February",0,true));
graphicValues.addDataLabel(graphicValues.new
DataLabel("March",0,true));
graphicValues.addDataLabel(graphicValues.new
DataLabel("April",0,true));
double[] series1 = { 4.49
, 3.47 , 1.50
, 1.71 };
graphicValues.addSeriesValues(series1);
double[] series2 = { 2.82
, 2.60 , 8.31
, 4.02 };
graphicValues.addSeriesValues(series2);
double[] series3 = { 3.49
, 4.52 , 7.94
, 9.06 };
graphicValues.addSeriesValues(series3); |
%>
<iframe name="drawFrame"
scrolling="yes" width="500" height="400"></iframe>
<!-- Call
the drawgraph servlet in post mode to generate the chart
image ->
<FORM action="servlet/JRDrawGraphServlet"
name="chartForm"
method=post target="drawFrame">
|
|
<input name="graph"
type="hidden" value="<%=
sGraphName %>">
<input name="xmldata" type="hidden"
value="<%= encodeURL(graphicValues.getXMLData(),"UTF-8"))
%>">
<input type="submit" value="<%=
Dictionary.getKey("*","Draw
Chart ",framework)%>"
> |
</FORM>
</BODY>
</HTML> |
|
JRDrawGraphServlet
input parameters |
|
graph
|
the graph administrative
object name |
xmldata
|
the xml text containing
the chart series values |
|
|
|
|
|